If the images do not show noticeable differences, it could be due to factors such as the original image’s characteristics, the magnitude of resizing, or the viewer’s display capabilities. Generally, for high-quality resizing, especially downscaling, Lanczos interpolation tends to provide superior results. If the images are small or the differences subtle, the choice of method may have less impact.
- Resampling: Resampling is the process of selecting a subset of the pixels from the original image to create the resized image. This method can result in loss of information or artifacts in the image due to the removal of pixels.
In Python, we can use the Pillow library for image resizing. Here is some example code for resizing an image using the Pillow library:
#resizing Image
from PIL import Image
# Open image
img = Image.open(‘../images/roseflower.jpeg’)
# Resize image
new_size = (200, 200)
resized_img = img.resize(new_size)
resized_img.save(“resized_image.jpg”)
We get the following result:
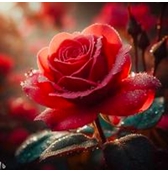
Figure 4.10 – Resized image (200*200)
In the preceding code, we first open an image using the Image.open() function from the Pillow library. We then define the new size of the image as a tuple (500, 500). Finally, we call the resize() method on the image object with the new size tuple as an argument, which returns a new resized image object. We then save the resized image using the save() method with the new filename.
Let’s see one more example of resizing images using Python. We first import the necessary libraries: os for file and directory operations and cv2 for image loading and manipulation:
import os
import cv2
We define the path to the image directory and get a list of all image filenames in the directory using a list comprehension:
# Define the path to the image directory
img_dir = ‘../Images/resize_images’
# Get a list of all image filenames in the directory
img_files = [os.path.join(img_dir, f) \
for f in os.listdir(img_dir) \
if os.path.isfile(os.path.join(img_dir, f))]
We define the new size of the images using a tuple (224, 224) in this example. You can change the tuple to any other size you want:
# Define the new size of the images
new_size = (224, 224)
We then resize the image as follows:
# Loop through all the image files
for img_file in img_files:
# Load the image using OpenCV
img = cv2.imread(img_file)
# Resize the image
resized_img = cv2.resize(img, new_size)
# Save the resized image with the same filename
cv2.imwrite(img_file, resized_img)
Here’s the output of the resized images in the relevant directory:
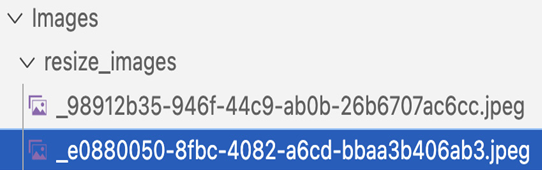
Figure 4.11 – Resized images in the directory
We loop through all the image files using a for loop. For each image file, we load the image using OpenCV (cv2.imread()), resize the image using cv2.resize(), and save the resized image with the same filename using cv2.imwrite().
The cv2.resize() function takes three parameters: the image to resize, the new size of the image as a tuple (width, height), and an interpolation method. The default interpolation method is cv2.INTER_LINEAR, which produces good results in most cases.
Resizing an image is a common preprocessing step in image classification and object detection tasks. It is often necessary to resize images to a fixed size to ensure that all images have the same size and aspect ratio, which makes it easier to train machine learning models on the images. Resizing can also help to reduce the computational cost of processing images, as smaller images require less memory and computing resources than larger images.
In summary, image resizing is the process of changing the dimensions of an image while preserving its aspect ratio. It is a common preprocessing step in computer vision applications and can be performed using interpolation or resampling techniques. In Python, we can use the Pillow library for image resizing.