Let us see the example LF for plant disease detection. In this code, we have created a rule to classify healthy and diseased plants, based on the color distribution of leaves. One rule is if black_pixel_percentage in the plant leaves is greater than the threshold value, then we classify that plant as a diseased plant.
The following are the two different types of plant leaves.
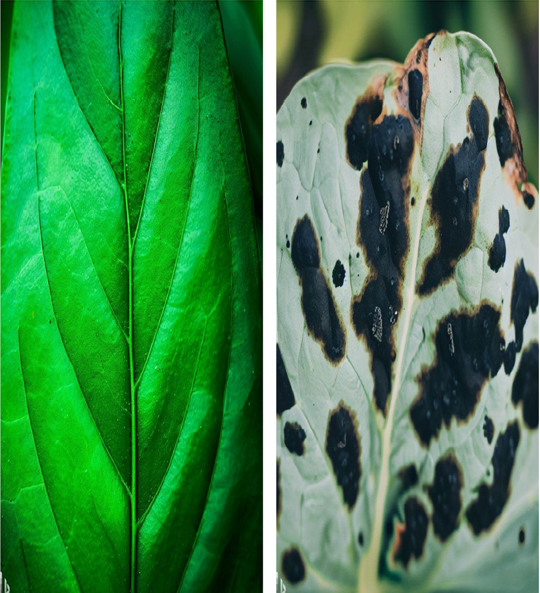
Figure 5.1 – Healthy and diseased plant leaves
We calculate the number of black color pixels in a leaf image and then calculate the percent of black pixels:
Percent of black pixels = count of black pixels in a leaf image/total number of pixels in a leaf image
We are going to use the rule that if the black pixel percent in a plant leave image is greater than the threshold value (in this example, 10%), then we classify that plant as a diseased plant and label it as a “diseased plant.”
Similarly, if the black pixel percentage is less than 10%, then we classify that plant as a healthy plant and label it as a “healthy plant.”
The following code snippet shows how to calculate the black pixel percentage in an image using Python libraries:
# Convert the image to grayscale
gray_image = cv2.cvtColor(resized_image, cv2.COLOR_BGR2GRAY)
This line converts the original color image to grayscale using OpenCV’s cvtColor function. Grayscale images have only one channel (compared to the three channels in a color image), representing the intensity or brightness of each pixel. Converting to grayscale simplifies subsequent processing:
# Apply thresholding to detect regions with discoloration
_, binary_image = cv2.threshold(gray_image, 150, 255,\
cv2.THRESH_BINARY_INV)
In this line, a thresholding operation is applied to the grayscale image, gray_image. Thresholding is a technique that separates pixels into two categories, based on their intensity values – those above a certain threshold and those below it. Here’s what each parameter means:
- gray_image: The grayscale image to be thresholded.
- 150: The threshold value. Pixels with intensities greater than or equal to 150 will be set to the maximum value (255), while pixels with intensities lower than 150 will be set to 0.
- 255: The maximum value to which pixels above the threshold are set.
- cv2.THRESH_BINARY_INV: The thresholding type. In this case, it’s set to “binary inverted,” which means that pixels above the threshold will become 0, and pixels below the threshold will become 255.
The result of this thresholding operation is stored in binary_image, which is a binary image where regions with discoloration are highlighted:
# Calculate the percentage of black pixels (discoloration) in the image
white_pixel_percentage = \
(cv2.countNonZero(binary_image) / binary_image.size) * 100
The cv2.countNonZero(binary_image) function counts the number of non-zero (white) pixels in the binary image. Since we are interested in black pixels (discoloration), we subtract this count from the total number of pixels in the image.
binary_image.size: This is the total number of pixels in the binary image, which is equal to the width multiplied by the height.
By dividing the count of non-zero (white) pixels by the total number of pixels and multiplying by 100, we obtain the percentage of white pixels in the image. This percentage represents the extent of discoloration in the image.
To calculate the percentage of black pixels (discoloration), you can use the following code:
black_pixel_percentage = 100 – white_pixel_percentage
Overall, this code snippet is a simple method to quantitatively measure the extent of discoloration in a grayscale image, by converting it into a binary image and calculating the percentage of black pixels. It can be useful for tasks such as detecting defects or anomalies in images. Adjusting the threshold value (in this case, 150) can change the sensitivity of the detection.
Let us create the labeling function to classify the plant as Healthy or Diseased, based on the threshold value of black_pixel_percentage in the leaf images, as follows.
# Define a labeling function to classify images as “Healthy”
@labeling_function()
def is_healthy(record):
# Define a threshold for discoloration (adjust as needed)
threshold = 10
# Classify as “Healthy” if the percentage of discoloration is below the threshold
if record[‘black_pixel_percentage’] < threshold:
return 1 # Label as “Healthy”
else:
return 0 # Label as “Diseased”
This LF returns labels 0 (a diseased plant) or 1 (a healthy plant) based on the black color pixels percentage in the image. The complete working code for this plant disease labeling is available in the GitHub repo.
In the next section, let us see how we can apply labels using image properties such as size and aspect ratio.